728x90
v-for는 객체, 배열, 컴포넌트와 함께 사용할 수 있다.
기본 사용 방법으로는 v-for"item in items" 의 형태
items는 순환하려는 배열 데이터의 출처를 의미하고,
item은 순환되는 요소의 별칭이다.
<html>
<head>
<title>test</title>
<meta charset="UTF-8">
<script src="https://unpkg.com/vue"></script>
</head>
<body>
<div id="app">
<ul>
<li v-for="item in items">{{item}}</li>
</ul>
<ul>
<li v-for="num in nums">{{num}}</li>
</ul>
</div>
<script type="text/javascript">
var app = new Vue({
el: '#app',
data: {
items: {
"a": "aa",
"b": "bb",
"c": "cc",
"d": "dd",
"e": "ee",
"f": "ff",
"g": "gg"
},
nums: [1, 2, 3, 4, 5]
}
})
</script>
</body>
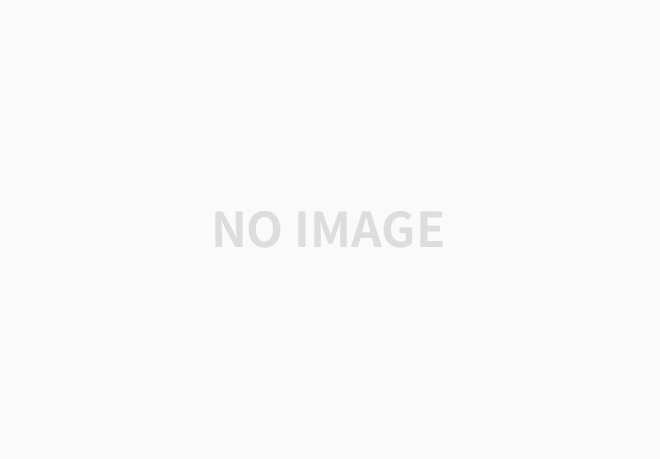
<span v-for="n in 5">✔</span>
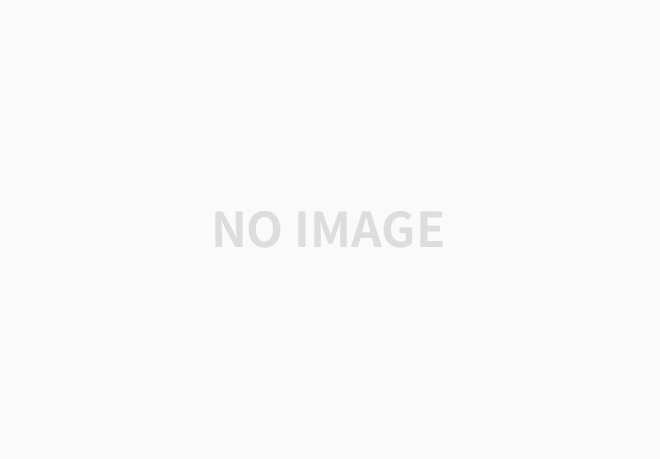
이런식으로도 사용할 수 있다.
이 외에
v-for="(value, index) in items"
v-for="(value, name, index) in items"
이렇게도 사용할 수 있다.
<html>
<head>
<title>test</title>
<meta charset="UTF-8">
<script src="https://unpkg.com/vue"></script>
</head>
<body>
<div id="app">
<ul>
<li v-for="(val, idx) in items">{{idx}} : {{val}}</li>
</ul>
<ul>
<li v-for="(val, name, idx) in items">[{{idx}}] {{name}} : {{val}}</li>
</ul>
</div>
<script type="text/javascript">
var app = new Vue({
el: '#app',
data: {
items: {
"a": "aa",
"b": "bb",
"c": "cc",
"d": "dd",
"e": "ee",
"f": "ff",
"g": "gg"
}
}
})
</script>
</body>
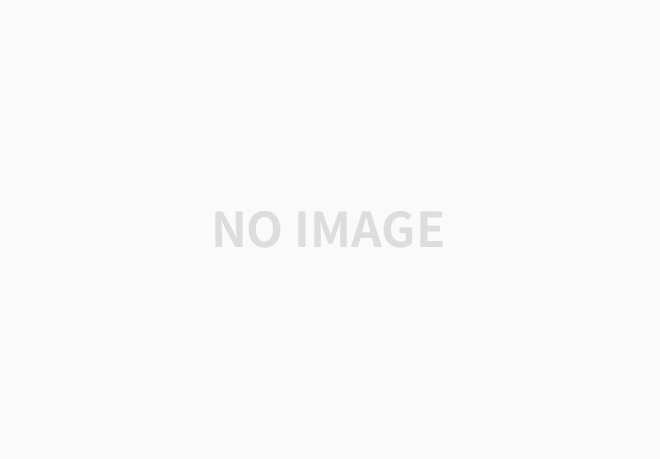
반응형
'VueJS' 카테고리의 다른 글
[VueJS] 컴포넌트 props와 리터럴속성 그리고 검증 (0) | 2021.01.27 |
---|---|
[VueJS] Component template requires a root element, rather than just text. (0) | 2021.01.24 |
[VueJS] v-if, v-else-if, v-else 사용시 알아둘 것 (0) | 2021.01.23 |
[VueJS] v-model, v-once (0) | 2021.01.21 |
[VueJS] computed와 methods (0) | 2021.01.20 |